The idea of components is not so new anymore, so I won’t have to spend a lot of time explaining what components are. Instead of that, I will spend most of the time talking about what they mean for us as an industry and highlighting the benefits and opportunities we get from using them.
How did we get to components?
To start things off I will contrast the way we were creating our frontends “in the old days” with the way we can do it now by simply thinking in components.
The picture below shows a basic example of the “old days” type of frontend code.
On the left side, there is the HTML file which defines the structure of a page, and on the right side, there is the CSS styling for the whole site.
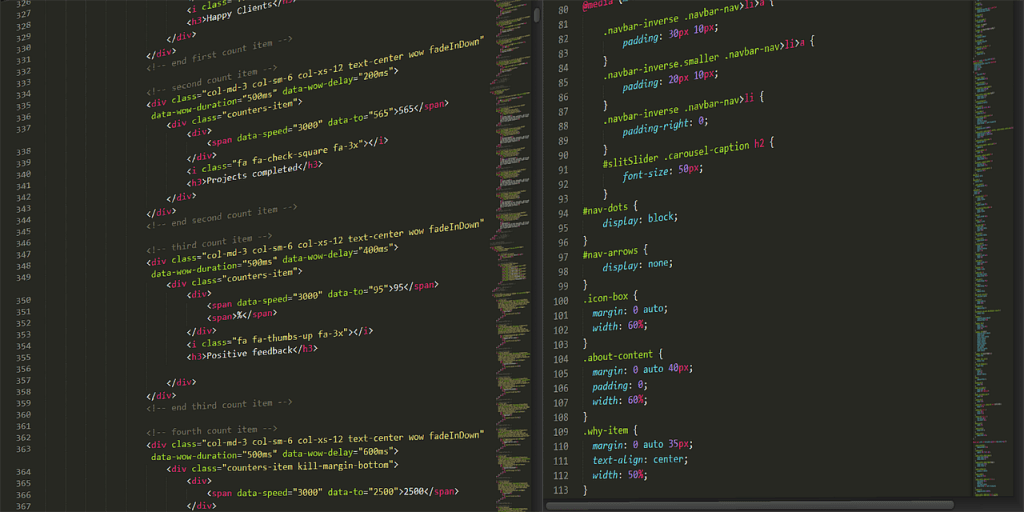
If we take a look at the right side of the screen we can take out a couple of different elements; there are some navigation related classes, an about-content, and a why-item.
Every page uses the same navigation and we would need to copy/paste it over and over again for every single page unless we separate it into a component.
We can encapsulate all of the structure, the styling and the logic of a particular component and include it inside the pages where they are needed.
--components
--|--navigation
--|--about-section
--|--why-item
--|-- ...
--pages
--|--homepage ( uses navigation and the why-item )
--|--about-us-page ( uses navigation and the about-content )
--|-- ...
In theory, our pages don’t necessarily have to have any HTML tags, they can be entirely made out of included components.
By structuring our code like this, we ensure two programming principles; we keep our code DRY (Don’t repeat yourself) and we get an easier to manage codebase because of the single source of truth representation of the components of our design system.
The many different flavors of components
All of the major .js frameworks (React, Angular, Vue) offer us a way to build and use components, each has its own particularities, but they all share the same idea of components as the main building blocks of an app or a website.
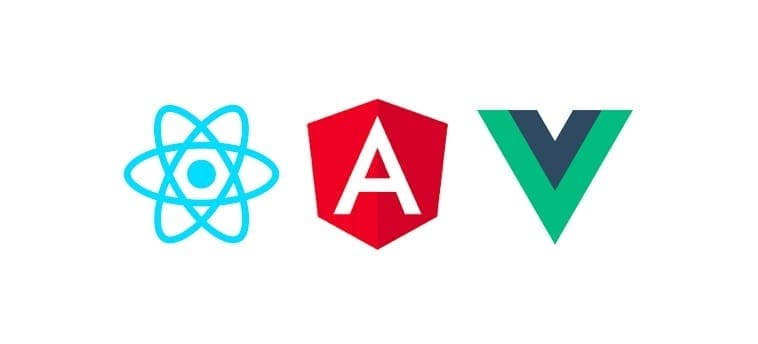
One thing that particularly interests me is the development of the specs and tools that enable us to create Web components which can then be used either within any of the frameworks or completely without one, which means we now have the ability to make and use framework agnostic components.
Web components can be especially beneficial to adopt for teams that work with different frontend stacks, because web components give everyone a way to easily understand and share each others code no matter the framework they are working with.
It can be worth noting that you could use the ideas of component-based architecture with most of the server-side templating engines like twig or blade as well. It is not the same, but we do get a lot of the benefits of thinking in components without any special tooling, but by applying some of the ideas of components to the architectural level alone.
That is exactly what we did with this particular site, it is built on top of WordPress but the frontend is written in twig with the help of Timber, while the pages are split into components and then used inside the views like this:
{% for career in careers %}
{% include "src/components/box-card/box-card.twig"
with {
tagline: career.tagline,
title: career.title,
text: career.short_text,
highlighted: career.highlighted,
link: career.link
}
%}
{% endfor %}
In my next post, I will go into details about how we organized our codebase based on components and extracted the used ideas into a boilerplate for all of our future WordPress based projects.
A block as a component
While talking about different flavors of components we need to mention Gutenberg blocks as well.
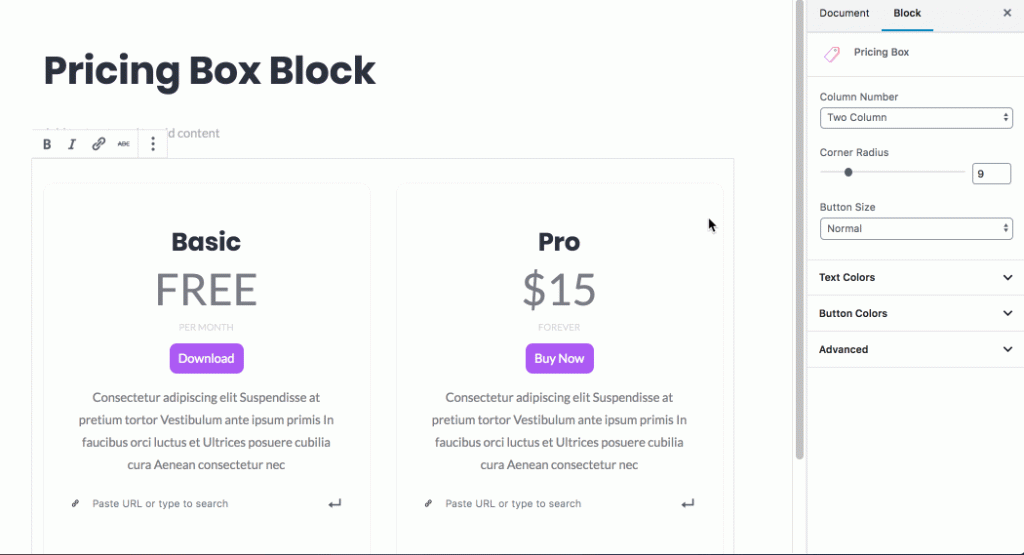
I would like to draw specific attention to this because it pushes the idea of components even further. What Gutenberg essentially is, when we look at it as someone who is a frontend developer, it is a way for us to give away the content input and the property states of our components out for a human user to directly fill in.
We are not feeding the content and the properties from within our own system, we are giving components to our users as a tool to build whatever they want with them.
Gutenberg isn’t the only tool pushing in that direction, for example with something like https://editorjs.io/ we could be seeing new ways to easily build very interesting custom content management systems around the idea of blocks.
The benefits of using components
By using component-based architecture for our apps and websites we open up a whole range of interesting benefits and opportunities that can improve the way in which we work and increase the quality of the products that we build.
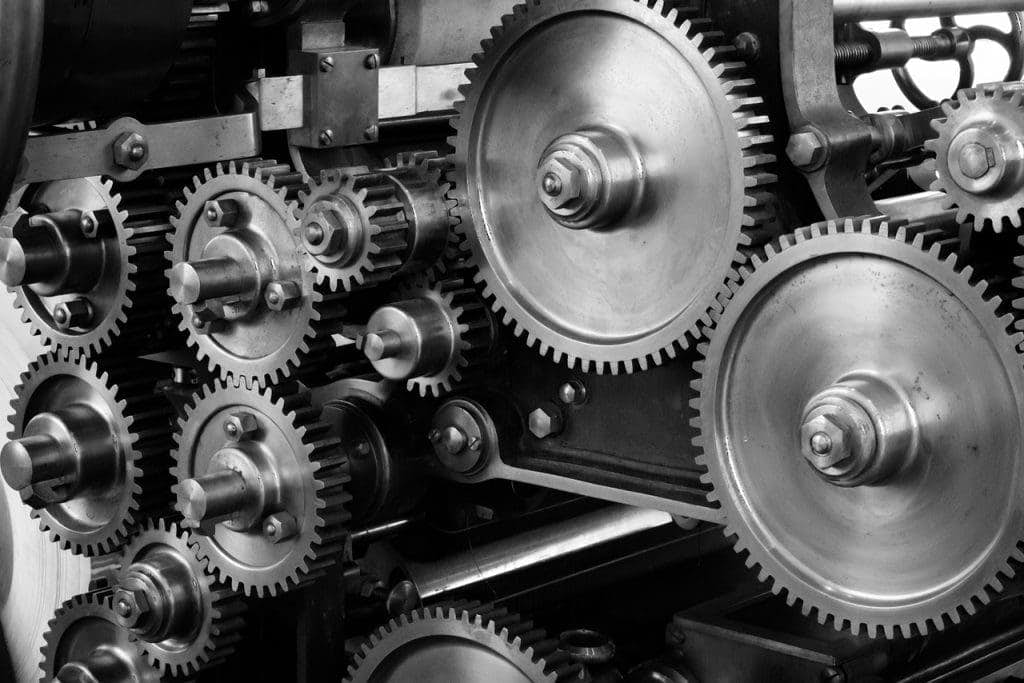
Code maintainability
A code base that is decoupled and isolated in logical chunks is a lot easier to test, debug and maintain. If you have a problem with the homepage slider, you know exactly where to look.
Existing systems can evolve and develop much faster if we can iterate over and optimize different parts in isolation without fearing to break something else in the process.
Code reusability
Apart of it being easier to maintain, as I mentioned at the beginning of this post, an existing code base organized in components can be helpful in every new project, giving you a part of the code that you can take out and modify for a new implementations to save us of doing the same things over and over again.
We can, of course, use third-party components (e.g. Ionic) from the start, without building any of them ourselves. But we have to keep in mind that we need to be able to easily change, extend and style the chosen components. It is often much better to have a particular solution built for a particular problem than to modify and override a general solution to a particular problem.
Pattern libraries and styleguide driven development
Once we have our interfaces divided into components, we can easily organize and present them with a pattern library (style guide). Two tools that I would recommend for doing so are https://storybook.js.org/ and
https://patternlab.io/.
Styleguide driven development suggests that we should develop our components in an isolated environment and only then implement them to particular pages where they are needed, I still haven’t really tried this approach, but having a pattern library is really beneficial by itself without necessarily using SDD.
Performance benefits
A couple of performance boosts can be easily achieved by using components, there is the obvious benefit of smaller total sizes of the files we send to our user. We can transfer all the files separately at the same time if we are using HTTP2 or easily bundle the needed components if not. We can load the critical render blocking parts at first and lazy load the rest later. We can even use components to easily set up progressive CSS rendering.
Easier collaboration
It would be kind of weird to see a couple of developers working on a single particular thing at the same time, they would be breaking each other’s work all the time. Furthermore, their total sum of productivity would be lower then if they worked on separate projects altogether. That is one of the reasons why we have Brooks’s law, which states that adding more developers to an existing project can sometimes make it move even slower.
The same issue does not apply when we are talking about components. If we divide up the workload to individual components we could have as many developers as there are components and have them all independently contribute to the overall project without messing up a piece of each others code.
Project planning and tracking
It is definitely beneficial to plan and track a project based on its features, all of the involved parties can have a shared understanding of set goals and the focus gets put on delivering fully functional, ready for production features.
However, considering components can also be of great help in making estimates, in tracking our time and in the end reviewing how good our starting estimates were. This is especially true for the front side of frontend development, cause most of the work of slicing (HTML/CSS) consists of creating components and placing them inside a layout. That way our tasks, can have a more direct correlation with completing the actual work.
Components in design
While there are many benefits of thinking in components when it comes to frontend development, it is beneficial to use the idea of components in design as well.
A component from the design perspective is a piece of the user interface, for example, a button, a gallery or a blog post. It is something your users can see, click on, or maybe even drag around. You can consider it as a single unit of the design that is used across a website, an app, or even a range of websites and apps of a single brand.
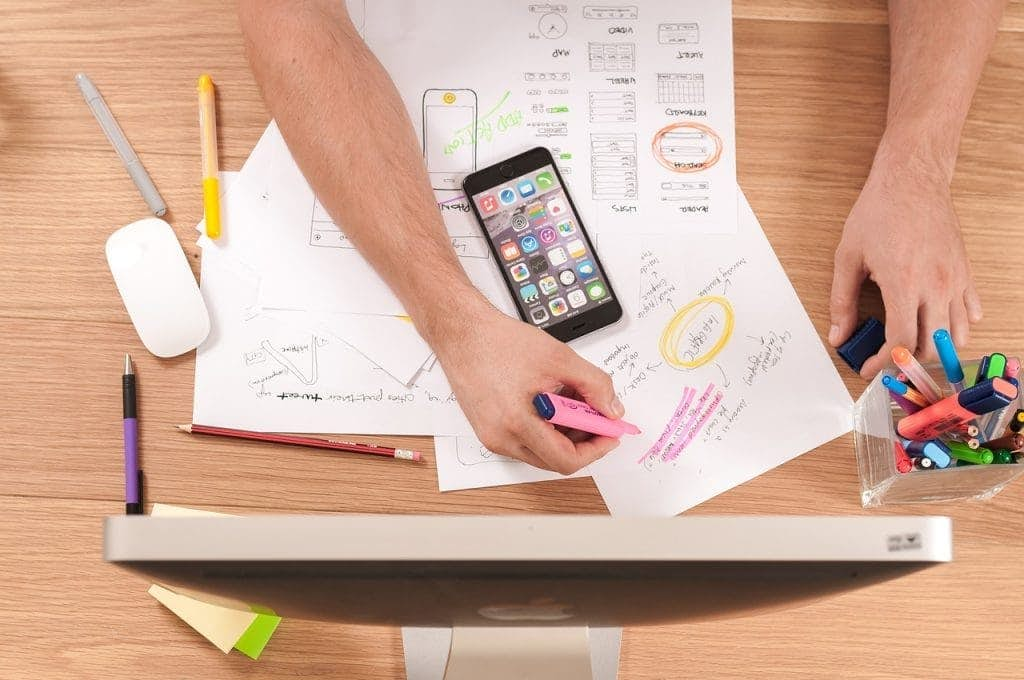
The designer can extract the small repeating patterns and organize them into a design system.
A design system split into components is distributed over to the frontend along with “quickly” made low fidelity wireframes and that is enough to get the development started, once the design system is in place, you can add additional features and pages directly from the wireframes.
Aside from helping with the speed of production and iteration, working in this way forces the designer to be more deliberate and thoughtful in defining the visual consistency representing the brand or a product.
Wrapping it up
That sums it up for now! We discussed how we got to components and we saw the various benefits of using them.
In my next post, I will get more practical and show examples of how are we using some of these ideas in our own development processes, here at Locastic.
Do you use components in your work, what is your experience with using them, I would really like to hear your thoughts as well, there is a comment section below, so feel free to share your ideas.
Till next time, happy coding.